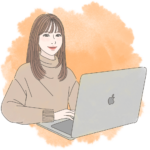
こんにちは、ウェブデザインパレットのmisaです。
Webサイトのトップページで、「Scroll Down」のボタンを見たことはありますか?
「Scroll Down」のボタンはアニメーションを使ったものが多く、ユーザーの視線を惹きつけ、ページへの興味を高めてくれる効果があります。
今回は、HTMLとCSS、Javascriptで、スクロールダウンのアニメーションを実装する方法について解説します。
コピペで使用できるので、ぜひみなさんのWebサイトでも試してみてくださいね。
テキストがくるくる回転するアニメーション
「SCROLL DOWN」の文字が円を形成して回転します。
矢印、マウスアイコンを中央に配置して、スクロールを示すアニメーションを設定しました。
JavaScriptを使用することで、テキストの内容や文字数を簡単に変更できます。
シンプルかつ動的で、ユーザーの注目を集めやすいデザインです。
矢印
コードを表示
<div class="container_01">
<a href="#" class="scroll-down_01">
<div class="circle-text_01"></div>
<div class="arrow_01"></div>
</a>
</div>
.container_01 {
margin: 0;
padding: 0;
height: 300px;
display: flex;
justify-content: center;
align-items: center;
background-color: #a5cbd7;
overflow: hidden;
}
.container_01 a:hover {
opacity: 0.7;
}
.scroll-down_01 {
position: relative;
width: 200px;
height: 200px;
color: #fff;
font-family: serif;
text-decoration: none;
}
.circle-text_01 {
position: absolute;
width: 100%;
height: 100%;
animation: rotate 20s linear infinite;
}
.circle-text_01 span {
position: absolute;
left: 50%;
font-size: 16px;
transform-origin: 0 100px;
}
.arrow_01 {
position: absolute;
top: 50%;
left: 50%;
width: 30px;
height: 50px;
transform: translate(-50%, -50%);
animation: scroll_01 3s infinite;
}
.arrow_01::before {
content: "";
position: absolute;
top: 0;
left: 50%;
height: 100%;
width: 1px;
background-color: #fff;
transform: translateX(-50%);
}
.arrow_01::after {
content: "";
position: absolute;
bottom: 2px;
left: 50%;
width: 12px;
height: 12px;
border-bottom: 1px solid #fff;
transform: translateX(-50%) rotate(-45deg);
}
@keyframes rotate {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
@keyframes scroll_01 {
0% {
transform: translate(-50%, -50%) translateY(-10px);
opacity: 0;
}
30% {
opacity: 1;
}
100% {
transform: translate(-50%, -50%) translateY(10px);
opacity: 0;
}
}
document.addEventListener("DOMContentLoaded", () => {
const text = "SCROLL DOWN ";
const repeatedText = text.repeat(2);
const circleText = document.querySelector(".circle-text_01");
const totalChars = repeatedText.length;
const anglePerChar = 360 / totalChars;
for (let i = 0; i < totalChars; i++) {
const span = document.createElement("span");
span.textContent = repeatedText[i];
span.style.transform = `rotate(${i * anglePerChar}deg)`;
circleText.appendChild(span);
}
});
矢印2
コードを表示
<div class="container_02">
<a href="#" class="scroll-down_02">
<div class="circle-text_02"></div>
<div class="arrow_02"></div>
</a>
</div>
.container_02 {
margin: 0;
padding: 0;
height: 300px;
display: flex;
justify-content: center;
align-items: center;
background-color: #b5d7a5;
overflow: hidden;
}
.container_02 a:hover {
opacity: 0.7;
}
.scroll-down_02 {
position: relative;
width: 200px;
height: 200px;
color: #fff;
font-family: serif;
text-decoration: none;
}
.circle-text_02 {
position: absolute;
width: 100%;
height: 100%;
animation: rotate 20s linear infinite;
}
.circle-text_02 span {
position: absolute;
left: 50%;
font-size: 16px;
transform-origin: 0 100px;
}
.arrow_02 {
position: absolute;
top: 50%;
left: 50%;
width: 30px;
height: 30px;
border-left: 1px solid #fff;
border-bottom: 1px solid #fff;
transform: translate(-50%, -50%) rotate(-45deg);
animation: pulse_02 2s infinite;
}
@keyframes rotate {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
@keyframes pulse_02 {
0%,
100% {
transform: translate(-50%, -50%) rotate(-45deg) scale(1);
}
50% {
transform: translate(-50%, -50%) rotate(-45deg) scale(1.1);
}
}
document.addEventListener("DOMContentLoaded", () => {
const text = "SCROLL DOWN ";
const repeatedText = text.repeat(2);
const circleText = document.querySelector(".circle-text_02");
const totalChars = repeatedText.length;
const anglePerChar = 360 / totalChars;
for (let i = 0; i < totalChars; i++) {
const span = document.createElement("span");
span.textContent = repeatedText[i];
span.style.transform = `rotate(${i * anglePerChar}deg)`;
circleText.appendChild(span);
}
});
マウス
コードを表示
<div class="container_03">
<a href="#" class="scroll-down_03">
<div class="circle-text_03"></div>
<div class="mouse_03"></div>
</a>
</div>
.container_03 {
margin: 0;
padding: 0;
height: 300px;
display: flex;
justify-content: center;
align-items: center;
background-color: #bfb7d5;
overflow: hidden;
}
.container_03 a:hover {
opacity: 0.7;
}
.scroll-down_03 {
position: relative;
width: 200px;
height: 200px;
color: #fff;
font-family: serif;
text-decoration: none;
}
.circle-text_03 {
position: absolute;
width: 100%;
height: 100%;
animation: rotate 20s linear infinite;
}
.circle-text_03 span {
position: absolute;
left: 50%;
font-size: 16px;
transform-origin: 0 100px;
}
.mouse_03 {
position: absolute;
top: 50%;
left: 50%;
width: 20px;
height: 30px;
border: 1px solid #fff;
border-radius: 10px;
transform: translate(-50%, -50%);
}
.mouse_03::before {
content: "";
position: absolute;
top: 5px;
left: 50%;
width: 1px;
height: 6px;
background-color: #fff;
transform: translateX(-50%);
animation: scroll_03 2s infinite;
}
@keyframes rotate {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
@keyframes scroll_03 {
0% {
top: 5px;
opacity: 0;
}
30% {
opacity: 1;
}
100% {
top: 15px;
opacity: 0;
}
}
document.addEventListener("DOMContentLoaded", () => {
const text = "SCROLL DOWN ";
const repeatedText = text.repeat(2);
const circleText = document.querySelector(".circle-text_03");
const totalChars = repeatedText.length;
const anglePerChar = 360 / totalChars;
for (let i = 0; i < totalChars; i++) {
const span = document.createElement("span");
span.textContent = repeatedText[i];
span.style.transform = `rotate(${i * anglePerChar}deg)`;
circleText.appendChild(span);
}
});
縦のスクロールバー
スクロールバーにアニメーションを設定して、スクロールを促しています。
「SCROLL」の文字を縦書きにしました。
JavaScriptを使用せず、HTMLとCSSのみで実装できます。
省スペースで、シンプル&スリムなデザインです。
ドットが上から下へ移動するアニメーション
コードを表示
<div class="container_04">
<div class="scrollbar-text_04"><span>scroll</span></div>
<div class="scrollbar_04"></div>
</div>
.container_04 {
height: 300px;
background-color: #cdb5b5;
}
.scrollbar-text_04 {
display: inline-block;
position: absolute;
bottom: 0;
padding: 10px 10px 110px;
color: #fff;
font-size: 14px;
font-family: serif;
line-height: 1;
letter-spacing: 0.2em;
text-transform: uppercase;
writing-mode: vertical-lr;
left: 50%;
transform: translateX(-50%);
}
.scrollbar_04 {
position: absolute;
left: 50%;
transform: translateX(-50%);
bottom: 1px;
}
.scrollbar_04::after {
content: "";
position: absolute;
bottom: 0;
left: 0;
width: 1px;
height: 100px;
background: #fff;
}
.scrollbar_04::before {
content: "";
position: absolute;
bottom: 0;
left: -4px;
width: 8px;
height: 8px;
border-radius: 50%;
background: #fff;
animation: circlemove 3s ease-in-out infinite,
cirlemovehide 3s ease-out infinite;
}
@keyframes circlemove {
0% {
bottom: 95px;
}
100% {
bottom: 0px;
}
}
@keyframes cirlemovehide {
0% {
opacity: 0;
}
50% {
opacity: 1;
}
80% {
opacity: 0.9;
}
100% {
opacity: 0;
}
}
縦線が上から下へ流れるアニメーション
コードを表示
<div class="container_05">
<div class="scrollbar-text_05">
<span>scroll</span>
</div>
<div class="scrollbar_05"></div>
</div>
.container_05 {
height: 300px;
background-color: #dad5a4;
}
.scrollbar-text_05 {
display: inline-block;
position: absolute;
bottom: 0;
padding: 10px 10px 110px;
color: #fff;
font-size: 14px;
font-family: serif;
line-height: 1;
letter-spacing: 0.2em;
text-transform: uppercase;
writing-mode: vertical-lr;
left: 50%;
transform: translateX(-50%);
}
.scrollbar_05 {
position: absolute;
left: 50%;
transform: translateX(-50%);
bottom: 1px;
}
.scrollbar_05::after {
content: "";
position: absolute;
bottom: 0;
left: 0;
width: 1px;
height: 100px;
background: #fff;
animation: liner 2.5s cubic-bezier(1, 0, 0, 1) infinite;
}
@keyframes liner {
0% {
transform: scale(1, 0);
transform-origin: 0 0;
}
30% {
transform: scale(1, 1);
transform-origin: 0 0;
}
70% {
transform: scale(1, 1);
transform-origin: 0 100%;
}
100% {
transform: scale(1, 0);
transform-origin: 0 100%;
}
}
その他
色々なデザインやアニメーションを実装してみました。
JavaScriptを使用せず、HTMLとCSSのみで実装できます。
円形の矢印
コードを表示
<div class="container_06">
<a href="#" class="scroll-down_06">
<div class="circle-arrow"></div>
Scroll Down
</a>
</div>
.container_06 {
height: 300px;
background-color: #a9cbc5;
}
.scroll-down_06 {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
text-align: center;
font-family: serif;
color: #fff;
font-size: 14px;
text-decoration: none;
}
.circle-arrow {
width: 40px;
height: 40px;
border: 1px solid #fff;
border-radius: 50%;
position: relative;
margin-bottom:12px;
margin-inline: auto;
animation: pulse 2s infinite;
}
.circle-arrow::after {
content: "";
position: absolute;
top: 50%;
left: 50%;
width: 10px;
height: 10px;
border-left: 1px solid #fff;
border-bottom: 1px solid #fff;
transform: translate(-50%, -50%) rotate(-45deg);
}
@keyframes pulse {
0% {
transform: scale(1);
}
50% {
transform: scale(1.1);
}
100% {
transform: scale(1);
}
}
マウスアイコン
コードを表示
<div class="container_07">
<a href="#" class="scroll-down_07">
<div class="mouse-icon"></div>
Scroll Down
</a>
</div>
.container_07 {
height: 300px;
background-color: #a6b0c6;
}
.scroll-down_07 {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
text-align: center;
font-family: serif;
color: #fff;
font-size: 14px;
text-decoration: none;
}
.mouse-icon {
width: 30px;
height: 50px;
border: 1px solid #fff;
border-radius: 20px;
position: relative;
margin-inline:auto;
margin-bottom: 6px;
}
.mouse-icon::before {
content: "";
width: 1px;
height: 8px;
position: absolute;
top: 8px;
left: 50%;
transform: translateX(-50%);
background-color: #fff;
border-radius: 2px;
animation: scroll 2s infinite;
}
@keyframes scroll {
0% {
opacity: 1;
transform: translate(-50%, 0);
}
100% {
opacity: 0;
transform: translate(-50%, 20px);
}
}
ウェーブ
コードを表示
<div class="container_08">
<a href="#" class="scroll-down_08 wavy-text">
<span style="--i:1">S</span>
<span style="--i:2">c</span>
<span style="--i:3">r</span>
<span style="--i:4">o</span>
<span style="--i:5">l</span>
<span style="--i:6">l</span>
<span style="--i:7"> </span>
<span style="--i:8">D</span>
<span style="--i:9">o</span>
<span style="--i:10">w</span>
<span style="--i:11">n</span>
<div class="wavy-text_arrow"></div>
</a>
</div>
.container_08 {
height: 300px;
background-color: #d0bdaa;
}
.scroll-down_08 {
position: absolute;
bottom: 40px;
left: 50%;
transform: translateX(-50%);
text-align: center;
font-family: serif;
color: #fff;
font-size: 14px;
text-decoration: none;
}
.wavy-text span {
position: relative;
display: inline-block;
animation: wave 2s infinite;
animation-delay: calc(0.1s * var(--i));
}
.wavy-text_arrow {
position: absolute;
top: 30px;
left: 50%;
width: 10px;
height: 10px;
border-left: 1px solid #fff;
border-bottom: 1px solid #fff;
transform: translate(-50%, -50%) rotate(-45deg);
animation: float 2s infinite;
}
@keyframes wave {
0%,
100% {
transform: translateY(0);
}
50% {
transform: translateY(-10px);
}
}
@keyframes float {
0%, 100% {
transform: translateY(-20%) rotate(-45deg);
}
50% {
transform: translateY(20%) rotate(-45deg);
}
}
グラデーションライン
コードを表示
<div class="container_09">
<a href="#" class="scroll-down_09">
<div class="gradient-line"></div>
Scroll Down
</a>
</div>
.container_09 {
height: 300px;
background-color: #f5f3f0;
}
.scroll-down_09 {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
text-align: center;
font-family: serif;
color: #809ee2;
font-size: 14px;
text-decoration: none;
}
.gradient-line {
width: 1px;
height: 80px;
background: linear-gradient(to bottom, transparent, #809ee2);
animation: extend 2s infinite;
margin-inline:auto;
}
@keyframes extend {
0%,
100% {
height: 0;
}
50% {
height: 80px;
}
}
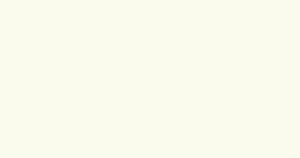
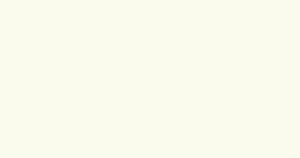
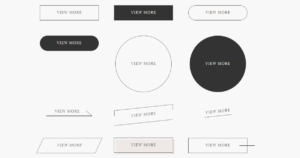
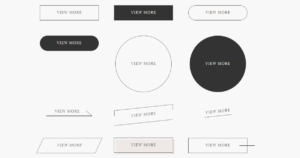
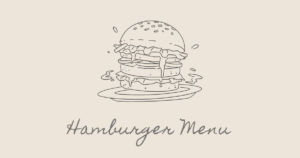
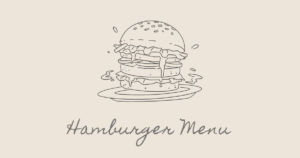
まとめ
今回は、、HTMLとCSS、Javascriptで、スクロールダウンのアニメーションを実装する方法について解説しました。
スクロールダウンアニメーションを取り入れることで、動きのあるWebページを作成することができます。
今後も、コピペで使えるアニメーションについての記事を追加していく予定なので、よかったらチェックしてみてください^^
では、また~✨